Collections in Java can be viewed as Collection Framework which is the most powerful feature. Collection Framework represents a hierarchy of interfaces and classes that is used to manage, handle and store groups of objects efficiently. It resides within java.util package. It wasn’t initially part of Java and was added later in J2SE 1.2.
Prior to Collection Framework, the legacy classes such as Dictionary, Vector, Stack, and Properties present in the framework, were used to store and manipulate groups of objects.
Java Collection Framework
It comprises of following :
- Interfaces
- Classes
- Algorithms
After the introduction of Generics, the collection framework became more powerful.
What are the advantages of the Collection Framework in Java?
- Increase in Performance: It provides support for high-performance data structures and algorithms such as dynamic arrays, linked lists, hash tables and trees which are highly efficient.
- Interoperability: It provides unified behaviour among collections as a result they can operate with one another
- Easy to Adopt and Use: It represents the hierarchy of interfaces and their implementation classes which can either be used directly or can be used to form a user-defined collection.
- Standard Array integration: It provides array integration into the collection framework.
- Reduces Programming Efforts: Each collection class is built upon some underlying data structure based on which it manipulates and works with objects. As a result, it provides several predefined methods for data searching, sorting, insertion, manipulation, and deletion which can be used as-is.
Hierarchy of Collection framework in Java
The below hierarchy resembles all the classes and interfaces that formulates Collection Framework architecture. These classes and interfaces reside within java.util package.
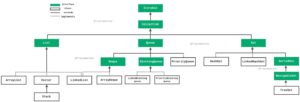
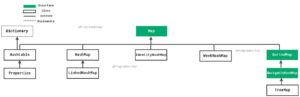
9 Key interfaces in Collection Framework
1. Collection Interface : It consists all the common methods that can be used by any collection object. It specifies behaviour specifically for List and Set. Therefore, it builds foundation for Collection Framework on which all collections work. It can be considered as root interface of collection framework. No class implements collection interface directly.
It provides core methods such as add(), remove(), isEmpty() and more, to work with collection objects.
Syntax:
public interface Collection<T> extends Iterable<T> { }
2. List Interface :
List Interface directly extends Collection. It represents ordered collection or sequence of objects. It specifies a type of data structure where duplicates are allowed and insertion order preserved. ArrayList, LinkedList, Vector and Stack are its implementing classes. Further, It supports implementation for legacy classes that are Vector & Stack which was introduced in Java 1.0 version.
Syntax :
public interface List<T> extends Collection<T> {}
3. Set Interface : A Set interface specifies a type of collection where duplicates are not allowed and insertion order is not preserved. It also extends collection interface. It allows null values and can store elements of different types. HashSet, LinkedHashSet, and TreeSet are the implementing classes of Set.
Syntax :
public interface Set<T> extends Collection<T> {}
4. SortedSet Interface : It is a child interface of Set. It doesn’t allow duplicates and represents group of elements in some sorting order. It accepts elements of same type that can be ordered in natural sorting order or user-defined order using Comparator.
Syntax:
public interface SortedSet<T> extends Set<T>
5. NavigivableSet Interface : It extends SortedSet. It defines various methods to traverse or navigate from one element to other in the given collection. TreeSet is the implementing class of NavigavbleSet.
Syntax:
public interface NavigableSet<T> extends SortedSet<T>{}
6. Queue Interface: It is a child interface of Collection. It represents a group of elements prior to processing or about to be processed in FIFO manner. In other words, it holds elements in a manner of first in first out order before processing. LinkedBlockingQueue and PriorityBlockingQueue are the classes that implements Queue interface.
Syntax:
public interface Queue<T> extends Collection<T>{}
7. Map Interface:
Map interface represents a group of objects as key value pair, not a group of individual objects. Therefore, Map and Collection interface are two different concepts, as a result, a separate hierarchy is followed in this case for collection Framework. HashMap,linkedHashMap, WeakHashMap, and Hashtable are the implementation class of Map interface.
Syntax:
public interface Map<K,V>
8. SortedMap Interface : It is a child interface of Map. It represents a group of elements in key value pairs where they are stored in some key sorting order.
Syntax:
public interface SortedMap<K,V> extends Map<K,V>{}
9. NavigableMap Interface: It is child interface of SortedMap.Simmilar to NavigableSet, it defines several methods for navigation or traversing purpose. TreeMap is the implementation class of this interface.
Syntax:
public interface NavigableMap<K,V> extends SortedMap<K,V>
Frequently Asked Questions:
1.What is Collection Framework in Java?
Collection Framework is an hierarchy of interfaces and classes to store and manipulate a group of elements efficiently.
2. Why we use Collection Framework in Java?
It provides several high performance classes that are built upon some underlying data structures, offering predefined methods which reduces programming efforts. Therefore, it is used to store and manipulate a group for elements efficiently.
3. Why we need Collection Framework in Java?
There are several limitations of arrays.
An array is not growable in nature ( one needs to define the size of an array at the time of decleration ) therefore, it’s fixed in size and can’t be increased or decreased as per requirement..
Secondly, it supports only elements of same types, as a result, it limits the store elements to the same type.
Lastly, it is not build upon some standard data structure, due to which, there are no predefined methods for data manipulation.
To overcome limitations of Arrays, collection is needed.