Both Break and Continue are jump statements in Java. These statements shift control from one part to another part of a program.
The break statement is mainly used to terminate the currently executing loop or block in which it’s encountered and move to next immediate program statement. On other hand, Continue statement only terminates the current iteration of the loop and hops to next iteration, without processing statements that come after it within the loop body. Sometimes, it requires If – condition to avoid Java’s unreachable statement error.
Difference between Break and Continue statement in Java in Tabular form
Basis | BREAK STATEMENT | CONTINUE STATEMENT |
---|---|---|
Definition | It is used to terminate the execution of the loop and shift the control over very next statement after the loop. It also terminates the sequence of cases of the switch statement | It is used to terminate the execution of the current iteration and jumps to the next iteration of the loop |
Control | It transfers control to the very first statement after the loop | It transfers control to the conditional expression of the loop. In other words, it goes back to the entry point of the loop and begins next iteration |
Termination | It causes termination of the loop | It causes termination of the current iteration of the loop, without processing other statements that come after it within the loop body |
Used with | It can be used with loops, switch statements and labels | It can only be used with loops |
Keyword | Break keyword is used for break statement in Java | Continue keyword is used for continue statement in Java |
Break Statement
Break statement is a jump statement that terminates a loop or a case sequence of switch statement in which it’s encountered.Therefore, it breaks the flow of a program.
It can be used to achieve the following functionality :
1) Escape from currently executing loop
2) Break the frequent execution of cases in Switch Statement
3) It can be used as Go-to Statement
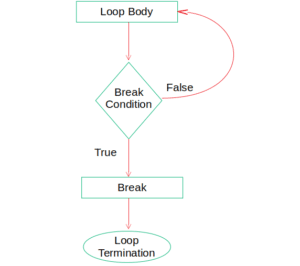
Java program to show working of break statement in a loop
public class BreakWithLoop { public static void main (String[]args) { for (int i = 1; i < 10; i++) { if (i == 5) { System.out. println ("Break Encountered ! \n ************* Terminating the loop ! "); break; } System.out.println ("Executing " + i + " times. "); } } }
Output:
Executing 1 times. Executing 2 times. Executing 3 times. Executing 4 times. Break Encountered ! ************* Terminating the loop !
- When break statement occurs in a loop, it forces immediate termination of that particular executing loop in which it’s encountered
- It skips all the remaining iteration and immediately moves control to next statement in the program
- In case of nested loop, if it is occurred in innermost loop, then it would be responsible for terminating inner loop only
- It is used in such situations when number of iterations are uncertain and therefore, it is companion with if statement
Java program to show working of break statement in Switch statement
public class BreakWithSwitch { public static void main (String[]args) { switch (2) { case 1: System.out.println ("case 1 :"); break; case 2:System.out.println ("case 2 :"); break; case 3:System.out.println ("case 3 :"); break; default:System.out.println ("nothing matched! :"); } }
Output:
case 3
When break statement triggers in a switch case, it terminates that case body in order to prevent from execution of later cases. In Switch statement, usually each and every case ends with a break statement to stop subsequent execution of cases.
Java program to show working of break statement with label
public class BreakWithLabel { public static void main (String[]args) { dog :{ cat :{ rat :{ System.out.println("Rat killed !"); break rat; } System.out.println("Cat killed !"); break cat; } System.out.println("Dog killed !"); break dog; } } }
Output:
Rat killed ! Cat killed ! Dog killed !
Java uses label and break statement in order to get some sort of functionality of go-to statement. In this, label works as a target point and break statement considers the label to jump out of that particular target point.
Continue Statement
It only terminates the current iteration of the loop and starts next iteration again, without processing statements that come after it within the enclosing loop body. It is used with looping statements only.
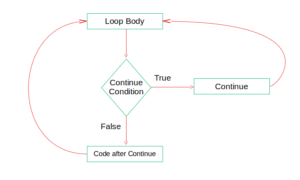
Java program to show working of continue statement in a loop
public class ContinueWithLoop { public static void main (String[]args) { for (int i = 1; i < 10; i++) { if (i <= 5) { continue; } System.out.println ("Executing " + i + " times. "); } } }
Output:
Executing 6 times. Executing 7 times. Executing 8 times. Executing 9 times.
When continue statement occurs in a loop, it forces immediate termination of the current iteration and jump back to beginning of the loop for next iteration. It skips only the current iteration and immediately moves control to next iteration in the loop.
It is important to have If – condition to avoid Java’s unreachable statement error.