Java supports various types of operators and most of these operators are grouped into four categories which are :
- Arithmetic operator
- Bitwise operator
- Relational operator
- Logical operator
In this article, we’ll be also discussing some of the special operators used in certain situations:
- Assignment Operator
- Unary Operator
- Ternary Operator
- Instance of Operator
An operator is used to perform operations on one, two or three operands, it can be either logical or mathematical operations, forming an expression that gives a new result. For example, a + b, where a and b are operands and + sign is an arithmetic operator.
Arithmetic Operator
Arithmetic operators are used to form a mathematical expression. This formed mathematical expression must consist of numeric operands. It cannot be used on other types such as char or boolean. In other words, it involves addition, subtraction, division and multiplication operations which operates on number operands i.e integer, float, and more.
There are primarily 5 types of arithmetic operators which are + (addition), – (subtraction), / (division), x (multiplication) and % (modulo) operator. Please refer below table for more. Other than that, there are two arithmetic unary operator, namely increment and decrement.
It involves the following operators:
Operators | Operations | Functionality |
+ | Addition | Add numbers |
– | Subtraction | Subtract number |
/ | Division | Divide number |
x | Multiplication | Multiply number |
% | Modulus | Get Remainder from division operation |
++ | Increment | Increment a number by 1 |
— | Decrement | Decrement a number by 1 |
+= | Addition Assignment | Add a number to a number at left side of assignment operator |
-= | Subtraction Assignment | Subtract a number to a number at left side of assignment operator |
/= | Division Assignment | Divide a number to a number at left side of assignment operator |
x= | Multiplication Assignment | Multiply a number to a number at left side of assignment operator |
%= | Modulus Assignment | Find remainder of division operation with a number to a number at left side of assignment operator |
Java Program to show the working of Arithmetic Operator
public class ArithmeticOperator { public static void main(String[] args) { int a = 10; int b = 5; System.out.println(" Addition = " + (a + b)); System.out.println(" Subtraction = " + (a - b)); System.out.println(" Division = " + (a / b)); System.out.println(" Multiplication = " + (a * b)); System.out.println(" Remainder = " + (a % b)); a++; System.out.println(" Increment = " + a); a--; System.out.println(" Decrement = " + a); System.out.println(" Addition assignment = " + (a += 6)); System.out.println(" Subtraction assignment = " + (a -= 6)); System.out.println(" Division assignment = " + (a /= 2)); System.out.println(" Multiplication assignment = " + (a *= 2)); System.out.println(" Remainder assignment = " + (a %= 5)); } }
Output:
Addition = 15 Subtraction = 5 Division = 2 Multiplication = 50 Remainder = 0 Increment = 11 Decrement = 10 Addition assignment = 16 Subtraction assignment = 10 Division assignment = 5 Multiplication assignment = 10 Remainder assignment = 0
Bitwise Operator
It performs all its operations only on integer types. As the name suggests, it works with the bits of an integer type values which is typical of 32 bits.
In other words, All the integer are represented by binary numbers on which bitwise operator works. An integer type can be negative and positive if the most significant bit of a given number represents 0 then it is a positive number or 1 then it is a negative number.
Below are the list of Bitwise operators :
Operator | Operations | Functionality | Example |
& | Bitwise AND | It performs AND operations among binary number. It is represented by & | 4 = 100 5= 101 4 :- 100 5 :-. &101 Output :- 100 |
| | Bitwise OR | It performs OR operations among binary number. It is represented by | | 4 = 100 5= 101 4 :- 100 5 :-. |101 Output :- 101 |
~ | Bitwise Unary not | It performs ones complement on binary number. It is represented by tilt sign (~) | 4 = 0000 …….100 4 :- 0000 …….100 ~ 1st complement = 0000….100 + 1 0000….101 Output = -5 |
^ | Bitwise exclusive OR | It performs exclusive OR operations on binary number. It is represented by ^ | 4 = 100 5= 101 4 :- 100 5 :-. ^101 Output :- 001 |
>> | Shift right | It shifts bits of binary number to the right as per the given bits that represents how much to shift | 4 = 00100 4 :- 00100 >> 1bit Output :- 00010 |
<< | Shift left | It shifts bits of binary number to the left as per the given bits that represents how much to shift | 4 = 00100 4 :- 00100 << 1bit Output :- 01000 |
>>> | Shift right Zero fill | It fills 0’s in a binary number from left in order to make a number positive or unsigned | 4 = 00100 4 :- 00100 >>> 1bit Output :- 00010 |
&= | Bitwise AND Assignment | Perform AND operation to a number with the number left side of assignment operator | Similar AND |
|= | Bitwise OR Assignment | Perform OR operation to a number with the number left side of assignment operator | Similar OR |
>>= | Shift right Assignment | Perform right shift operation mentioned at right side on a number at left side of assignment operator | Similar Shift right |
>>>= | Shift right Zero fill Assignment | Perform unsigned right shift operation and fills 0’s in most significant bits mentioned at right side on a given number at left side of assignment operator | Similar Shift right Zero fill |
<<= | Shift left assignment | Perform left shift operation mentioned at right side on a number at left side of assignment operator | Similar Shift left |
Java program to show the working of Bitwise operators
public class BitwiseOperator { public static void main(String[] args) { int a = 4; int b = 5; System.out.println(" AND = " + (a & b)); System.out.println(" OR = " + (a | b)); System.out.println(" Complement = " + (~a)); System.out.println(" XOR = " + (a ^ b)); System.out.println(" Right Shift = " + (a >> 1)); a = 4; System.out.println(" Left Shift = " + (a << 1)); a = 4; System.out.println(" Unsigned Right Shift = " + (a >>> 1)); } }
Output:
AND = 4 OR = 5 Complement = -5 XOR = 1 Right Shift = 2 Left Shift = 8 Unsigned Right Shift = 2
Relational Operator
It is used to define a relationship between two operands by determining their equality and ordering. It always returns a boolean value. It can be mostly seen in loops and If statement.
It includes equality and inequality that can be applied to characters, numeric type and more, whereas ordering operators can only be applied on the numeric type.
Operator | Operations |
== | Equality check |
!= | Inequality check |
>= | Greater than equal to check |
<= | Smaller than equal to check |
> | Greater than to check |
< | Smaller than to check |
Java program to show the working of Relational operator
public class RelationalOperator { public static void main(String[] args) { int x = 0; boolean result; result = x==0; System.out.println("Equality Operator = "+result); result = x!=1; System.out.println("Inequality Operator = "+result); result = x>=0; System.out.println("Greater than equal to Operator = "+result); result = x<=0; System.out.println("Smaller than equal to Operator = "+result); result = x<1; System.out.println("Smaller Operator = "+result); result = x>-1; System.out.println("Greater Operator = "+result); } }
Output:
Equality Operator = true Inequality Operator = true Greater than equal to Operator = true Smaller than equal to Operator = true Smaller Operator = true Greater Operator = true
Logical Operator
It combines two or more relational expressions together in which each expression return a boolean value. These boolean values are the values on which logical operators work and again a true or false is evaluated for decision making.
Operator | Operations | Functionality |
& | Logical AND | If condition A and B are evaluated as true then only it returns true |
| | Logical OR | Either A or B is evaluated as true then it would return true |
^ | Logical XOR ( Exclusive OR) | If condition A evaluates different from B them it would return true. In other words, if A condition returns true then B should be false in order to evaluate true |
|| | Short-circuit OR | It only evaluates first or left expression, if it returns true then it would return true, without evaluating second or right expression |
?: | Ternary if then else | If a condition is evaluated as true, then it would return first statement followed by ? Symbol. Otherwise, second statement would be returned |
&& | Short-circuit AND | It evaluates first expression, if it returns true then it would evaluate second condition if it returns true, then final outcome will be true |
! | Logical Unary not | If condition A is evaluated as false then it would return true |
Java program to show the working of Logical operator
public class LogicalOperator { public static void main(String[] args) { int x = 10, y = 20; System.out.println("AND operator = " + ((x > 9) & (y > 9))); // 10>9 & 20>9, since both are true result is true System.out.println("OR operator = " + ((x < 9) | (y > 9))); // 10<9 | 20>9, since left one is true result is true System.out.println("XOR operator = " + ((x < 9) ^ (y > 9))); // 10<9 ^ 20>9, since both are different one is false and other one is true result is true System.out.println("Short-Circuit AND operator = " + ((x < 9) && (y > 9))); //10<9 && 20>9, only left one is evaluated which is false System.out.println("Short-Circuit OR operator = " + ((x < 9) && (y > 9))); //10<9 && 20>9, only left one is evaluated which is false System.out.println("Ternary Operator AND operator = " + ((x > y) ? 1 : 0)); //10>20? 1:0, expression before ? is false, which would return second statement after ? that is 0 System.out.println("Not Equal operator = " + (!(x > y))); // 10>20, since it is false and when this evaluated false again complemented it returns true } }
Output:
AND operator = true OR operator = true XOR operator = true Short-Circuit AND operator = false Short-Circuit OR operator = false Ternary Operator AND operator = 0 Not Equal operator = true
Assignment Operator
It is used to assign a value to an operand. The operand must be compatible with the type of expression.
It assigns a value of right hand side operand to another operand presenting at left side.
int a =10 ;
Unary Operator
Unary Operator is a single operand operator. It can be defined as an operator that operates on only a single operand and performs operations such as increment, or decrement on a variable. It uses operators ranging from arithmetic to logical to perform operations on individual variables i.e +,-,!.

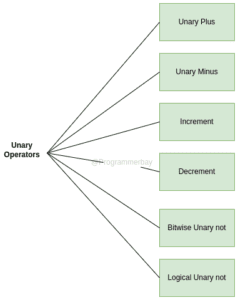
Operators | Operations | Example |
+ | Unary Plus provides positive value for the arithmetic operand | +x |
– | Unary Minus provides negative value for the arithmetic operand | -x |
++ | Increment Operator increases the operand value by 1 | x= x+1 (pre-increment) : ++x; (post-increment) : x++; |
— | Decrement Operator decreases the operand’s value by 1 | x=x-1 (pre-decrement) : —x; (post-decrement) : x–; |
! | Logical Unary Not negates inverse the operand’s logical value | !x |
~ | Bitwise Unary Not performs one’s complement on binary number | ~x |
Java program to show the working of Unary Operator
public class UnaryOperator { public static void main(String[] args) { int x = 4; int result; result= -x; System.out.println("Unary Minus operator :: "+ result); result = +x; System.out.println("Unary Plus operator :: "+ result); System.out.println("Increment operator :: "+ ++x); System.out.println("Decrement operator :: "+ --x); System.out.println("Logical Not operator :: "+ !(x < 10)); System.out.println("Bitwise Not operator :: "+ ~(x)); } }
Output:
Unary Minus operator :: -4 Unary Plus operator :: 4 Increment operator :: 5 Decrement operator :: 4 Logical Not operator :: false Bitwise Not operator :: -5
Ternary Operator
Ternary operator operates on three operands which are conditional expression, truthy statement, and falsy statement. If an expression is turned out to be truthy, statement 1 would be executed otherwise, statement 2 which is falsy, would be executed.
It can be used in place of certain if -then-else statement situation. It is represented by ?: operator.
expression1 ? statement 1 : statement 2
Java program to show the working of ternary operator
public class TernaryOperator { public static void main(String[] args) { Scanner inputReader = new Scanner(System.in); System.out.print("Please enter your age : "); int voterAge = inputReader.nextInt(); String msg = voterAge < 18 ? "you are not eligible" : "You can vote"; System.out.println(msg); } }
InstanceOf Operator
InstanceOf operator can be defined as an operator that checks whether an object belongs to an instance of a class or not. It is a binary operator that compares instance type, if the given object is equivalent to an instance of a targeted class, it would return true otherwise false. It also applies to subclass and interface.
Java program to show the working of instanceOf operator
public class InstanceOfOperator { public static void main(String[] args) { Integer x = 10; if(x instanceof Number){ System.out.println("Integer is Subclass of Number class"); } } }
Output :
Integer is Subclass of Number class