An object without having reachable references is considered to be as unreachable which is later collected by a garbage collector in Java. A Garbage collector is responsible for destroying these objects in order to free the occupied heap memory. It is automatically invoked by JVM.
Programmatically, it’s not possible to force garbage collection. However, one can only request to JVM to perform garbage collection. There are two ways to make a request to Java Virtual Machine in order to execute garbage collector explicitly or force it for Garbage Collection:
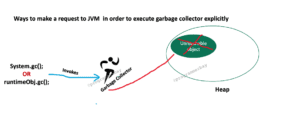
Calling Garbage Collector Using System Class
This class support rich methods that deals with standard input and output, loading files and libraries and so on. It falls under Java.lang package. It also provides a method named gc() to request JVM to make an effort to run a garbage collector.
Syntax:
System.gc();
Since it is a static method, therefore, it is invoked by using the class name.
Program:
public class ExplicitGarbageCollectorCall { @Override protected void finalize () throws Throwable { System.out.println ("I am releasing resources !"); } public static void main (String[]args) { ExplicitGarbageCollectorCall obj = new ExplicitGarbageCollectorCall (); // object is created obj = null; // now object is unreachable System.gc (); } }
Output:
I am releasing resources!
Calling Garbage Collector By using runtime class
Runtime class is a singleton class that deals with the interaction of an application with JRE. It consists only a single instance and getRuntime method is used to get that instance of the class.
1) Get the singleton instance of Runtime class using factory method.
Runtime obj = Runtime.getRuntime();
Since getRuntime() is a static method, therefore, the class name is used to invoke it.
2) Using this object, we now call any number of instance methods that come under Runtime class.
obj.gc();
Runtime class also comes with some useful methods these are totalMemory() and freeMemory(). As name suggests, totalMemory() returns total memory space whereas freeMemory() returns free memory space.
Program:
public class ExplicitGarbageCollectorCall { @Override protected void finalize () throws Throwable { System.out.println ("I am releasing resources !"); } public static void main (String[]args) { ExplicitGarbageCollectorCall obj = new ExplicitGarbageCollectorCall (); // object is created obj = null; // now object is unreachable System.gc (); Runtime rt = Runtime.getRuntime (); rt.gc (); System.out.println ("Total memory space = " + rt.totalMemory ()); System.out.println ("Free memory space = " + rt.freeMemory ()); } }
Output:
I am releasing resources! Total memory space = 64487424 Free memory space = 63527240
There is no difference between gc() method of System and Runtime class, both are committed for doing the same task which is destruction of the unreachable object. System.gc() is an easier way to invoke GC explicitly than runtime class.
Frequently Asked Questions
Q1. Is it possible to force garbage collection in Java ?
No, it’s not possible to force garbage collection in Java. One can only request to Java Virtual Machine to perform garbage collection. Although, it doesn’t guarantee immediate execution of the request.