In Java, data types define what type and range of data a variable can hold. Java supports strictly typed datatypes that are distinct from C/C++ datatypes whose size varies with the system architecture but in case of Java, they are of fixed size irrespective of architecture.
This is why strictly typed datatypes provides better performance and robustness over C/C++.
Java Primitive Data Types
Java is a strongly typed language in which all variables have a datatype. A datatype signifies what values a variable can store and what are the operations on the data can be performed.
Since, Java is statically typed that ensures a variable’s type definition at compile-time, before using that respective variable.
Syntax :
int sum = 23;
In the above example, “sum” is a variable, and 23 is its value. “int” is a data type that is telling the compiler, that “sum” can hold only those values that are considered to be int type, which is integer values, not decimal. If you try to assign 23.89 to it, then the compiler would throw exception.
There are two types of data types: primitive and reference type. We will discuss reference type later.
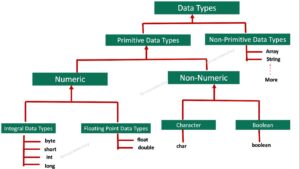
- Numeric data types : These datatypes represent numbers. It can be further have integral data types i.e 6 which doesn’t have any decimal point and floating point data type which are represented by decimal numbers i.e 6.5.These are signed types as it can be represent in positive and negative value.
- Integral primitive data types: It resembles a whole number or a number without having decimal point. This consists byte, short, int and long.
- Floating point primitive data types: It resembles numbers having fractional parts. This consists float and double.
- Non-numeric primitive data types: These represent non-numeric data. It can also be divided into two parts character and Boolean data type.
- Character data type: It represents a character. This consists char
- Boolean data type: It represents true or false.This consists boolean.
Java data types range, default value and size in tabular form
Data types | Default Value | Size | Supported Range |
---|---|---|---|
byte | 0 | 1 byte ( 8 bits ) | -128 to 127 |
short | 0 | 2 bytes ( 16 bits ) | -32,768 to 32,767 |
int | 0 | 4 bytes ( 32 bits ) | -231 to 231 - 1 |
long | 0L | 8 bytes ( 64 bits ) | -263 to 263 - 1 |
float | 0.0f | 4 ( 32 bits) | Beyond scope |
double | 0.0d | 8 (64 bits) | Beyond scope |
boolean | false | 1 bit | true or false |
char | \u0000 | 2 bytes ( 16 bits ) | 0 to 65,535 |
What are the Primitive Data Types ?
Integers: byte, short, int, and long fall into this group and stores integer values ( both negative and positive). For example, 23 and 34.
Characters: char falls into this group as it represents a symbol of characters. For example, ‘0’ and ‘A’.
Floating-point: float and double are grouped into this class where all floating values represented. For example, 23.34 and 34.34.
Boolean: a Boolean type can have true or false values which prove handy for decision making.
Java Numeric Data Types
Integral Data types or Integers:
Byte (Read the topic in brief)
Byte is an 8-bit size that is capable of storing signed data of 28-1 ( 28-1 = 256), ranging from -128 to 127. It is used to work on a stream of data from file or network. It is the smallest Integer data type and declared by byte keyword.
Point to remember:
- Size : 8-bit
- Default value : 0
- Range : from -128 to 127
Program example:
public class ByteExamples { public static void main(String[] args) { byte byteVar = 23; System.out.println("an example of byte :: "+ byteVar); } }
Short (Read the topic in brief)
Short comes with 16-bit data storing capability to work with image processing. It is declared by short keyword.
Point to remember:
- Size : 16-bit
- Default value : 0
- Range: from -32,768 to 32,767
Program example:
public class ShortExamples { public static void main(String[] args) { short shortVar = 100; System.out.println("an example of short :: "+ shortVar); } }
Int (Read the topic in brief)
A 32-bit datatype that is commonly used to work with arrays and control statements. It provides enough range to perform a small arithmetic operation. It is declared by int keyword.
Point to remember:
- Size : 32-bit
- Default value : 0
- Range: from -231 to 231-1.
Program example:
public class IntegerExamples { public static void main(String[] args) { int num = 100; System.out.println("an example of int :: "+ num); } }
long (Read the topic in brief)
It is signed 64-bit datatype and largest among all the integer datatypes. It represents a quite large number. It is declared by long keyword.
Point to remember:
- Size : 64-bit
- Default value : 0L
- Range: from -263 to 263-1.
Program example:
public class LongExamples { public static void main(String[] args) { long lonNum = 24434; System.out.println("an example of long :: "+ lonNum); } }
Floating Point Data types :
Float (Read the topic in brief)
It is 32-bit sized datatype for storing real numbers having fractional precision. It represents single precision values. It is declared by float keyword.
Point to remember:
- Size : 32-bit
- Default value : 0.0f
- Specifies single precision values
Program example:
public class FloatExample { public static void main(String[] args) { float num = 3.4f; System.out.println("an example of float :: " + num); } }
Double (Read the topic in brief)
It is 64-bit sized datatype for storing real number having fractional precision. It is useful when the accuracy of the result required. It is declared by double keyword.
Point to remember:
- Size : 64-bit
- Default value : 0.0d
- specifies single double values
Program example:
public class DoubleExample { public static void main(String[] args) { double num = 3.4; System.out.println("an example of double :: " + num); } }
Java Non – Numeric Data Types
Character:
Char (Read the topic in brief)
It is 16-bit sized datatypes for storing a symbol of the character set. Java uses Unicode to represent characters. It is declared by char.
Point to remember:
- Size : 16-bit
- Default value : ‘\u0000’
- Range: from 0 to 65,535
Program example:
public class CharacterExample {
public static void main(String[] args) {
char chr = 'A';
System.out.println("an example of char :: " + chr);
}
}
Boolean:
boolean (Read the topic in brief)
Another primitive that Java supports is Boolean. It can have either true or false value. It is quite a helpful datatype for decision making.
Point to remember:
- Size : 1-bit
- Default value : false
- Possible values: true or false
Program example:
public class BooleanExamples {
public static void main(String[] args) {
boolean bool = true;
System.out.println("an example of boolean :: "+ bool);
}
}
Java Program to show the representation of primitive data types
Program:
public class Sample { public static void main(String[] args) { byte a=10; short b=2; int c =6; long d=20; float e =21; long f= 24; char i= 'c'; System.out.println(a); System.out.println(b); System.out.println(c); System.out.println(d); System.out.println(e); System.out.println(f); System.out.println(i); } }
Output:

Non-Primitive Data Type
A non primitive data types are also known as reference types as these types points to a memory address where actual data stores. It gets memory in heap.
Arrays, Strings are some examples of reference type.