The program demonstrate deadlock situation between two threads. When two threads try to access each other’s resources but end up with waiting for other thread to release the respective locks that are acquired by them. Since, no one releases the lock, a problem of deadlock arises.
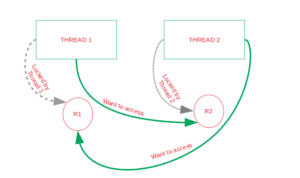
Java Program to Create Deadlock Between Two Threads
Program:
// Client class class Client { public synchronized void clientMethod(Programmer p){ System.out.println(Thread.currentThread().getName()+" is Trying to execute method of Programmer : "); try { Thread.sleep(5000); } catch (InterruptedException e) { e.printStackTrace(); } // Waiting for releasing lock of programmer p.display(); } public synchronized void display() { System.out.println("I am invoking display method of Client "); } } //Programmer Class class Programmer { public synchronized void programmerMethod(Client c){ System.out.println(Thread.currentThread().getName()+" is Trying to execute method of client : "); try { Thread.sleep(5000); } catch (InterruptedException e) { e.printStackTrace(); } // Waiting for releasing lock of client c.display(); } public synchronized void display() { System.out.println("I am invoking display method of Programmer "); } } public class Main { public static void main(String[] args) { Programmer programmerObj = new Programmer(); Client clientobj = new Client(); Thread threadOne = new Thread() { public void run() { programmerObj.programmerMethod(clientobj); } }; Thread threadTwo = new Thread() { public void run() { clientobj.clientMethod(programmerObj); } }; threadOne.start(); threadTwo.start(); } }
Output:
Explanation:
Client thread acquires lock over Programmer thread’s resources (that are methods and attributes of programmer class). Similarly, Programmer thread obtains lock over Client’s resources. After acquiring a lock, both went to sleep for 5 seconds.
Since Client thread has Programmer’s resources lock and on the other hand, Programmer thread has Client’s resources lock. When the Client thread tries to access ‘display’ method, it figures out that the lock of client resources is acquired by Programmer. As a result, both are ended up in a deadlock situation.