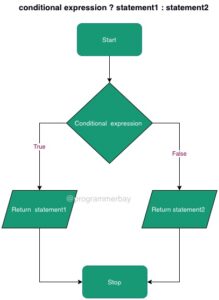
- condition
- truthy statement
- falsy statement
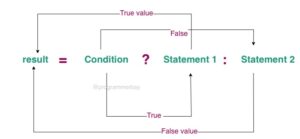
expression1 ? statement 1 : statement 2
String result = ( x == y ) ? “a true statement ” : “ a false statement”
When the Java compiler reaches this statement, if expression (x == y) gets evaluated as true, then “a true statement ” would be returned, if the expression is determined as false, then “a false statement” ( the statement after would be returned) .
At last, the returned statement would be assigned to the respective variable.
Typically, if-then-else situation can be replaced by a ternary operator.
if (voterAge < 18) { System.out.println("You're not eligible for voting"); } else { System.out.println("You can vote"); }
System.out.println(voterAge < 18 ? "You're not eligible for voting" : "You can vote");
Java program to show the working of ternary operator
public class TernaryOperator { public static void main(String[] args) { Scanner inputReader = new Scanner(System.in); System.out.print("Please enter your age : "); int voterAge = inputReader.nextInt(); String msg = voterAge < 18 ? "You're not eligible for voting" : "You can vote"; System.out.println(msg); } }
Please enter your age : 23 You can vote
Please enter your age : 12 You're not eligible for voting
Where to use the Ternary Operator?
Ternary operator returns a value that is mandatorily required to assign to a variable or used. In contrast, if-then-else is a control statement that controls the flow of a program and doesn’t return any value. Otherwise, the compiler would give a time exception.
Ternary operator can be used in place of if-then-else statement where a variable requires to assign value based on the condition.
Nested Ternary Operators
public class TernaryOperator { public static void main(String[] args) { Scanner inputReader = new Scanner(System.in); System.out.print("Please enter your age : "); int voterAge = inputReader.nextInt(); String msg = voterAge < 18 ? (voterAge<13)? "you are a baby": "you are teenager" : "You can vote"; System.out.println(msg); } }
The above code can be explained as,
- If entered age is greater than 18, then statement after “:” would be evaluated and a falsy statement returned, which is “you can vote”.
- If entered age is lower than 18, then another ternary operator, followed by ?, would be evaluated
- If inner condition which is (voterAge<13) evaluated as true, then “you are a baby” would be printed
- Otherwise, falsy statement of nested ternary operator would be executed.