In this article, we’ll be discussing what is use of inheritance and why multiple inheritance is not supported in Java .
One of the main features of object-oriented programming is Inheritance. In Java, Inheritance can be defined as a way by which a class acquires properties from another class.
A class which inherits properties from another class is known as subclass or child class, while the class which is inherited, is termed as superclass or parent class. extends keyword is used in order to inherit the properties of another class.
Syntax:
class SubClass extends ParentClass { // Attributes and behavior }
As and when, a class uses extends keyword, it can access and include all data members and member functions of the inherited class . However, a child class can never access and inherit the private members of parent class.
Why we use Inheritance in Java ?
Inheritance provides a number of features:
- Code Reusability:
Code reusability means an existing class can be reused in other class, specifically for similar type of classification.
- Ease to add new feature:
One can provide new functionality to a new class by using the existing class without changing it.
- Overriding
This provides the freedom of having methods with the same signature as in parent class but with meaningful implementation.
Why Multiple Inheritance is Not Supported in Java?
Multiple Inheritance is a type of inheritance in which a class extends two or more classes.
Unlike C++, Java doesn’t support multiple inheritance.
The reason because multiple inheritance raises ambiguity problem which creates the possibility of inheriting multiple copies or generating multiple paths of same data for child class.
In other words, a compiler can’t able to determine which member to choose if inheriting classes have common members. This problem is termed as Diamond Problem.
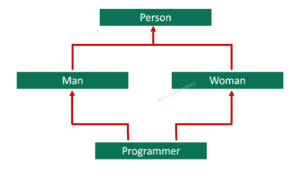
For example, suppose a class represents Person having getGender() method and this Person Class is inherited by Man and Woman Class. Since both have inherited getGender() method from Person Class.
However, if Man and Woman Class is further extended by another class named Programmer. As a result, the Programmer Class would get two copies of “getGender()” method which leads to ambiguity for compiler. Meaning, a compiler won’t be able resolve method call at runtime.
Program to show the concept of Inheritance
class A { int a; A() // constructor { a=10; } void display() // method { System.out.println("I am here with "+ a); } } class B extends A{ // inheriting void show() { display(); } } public class Inheritanceuseing { public static void main(String[] args) { B obj = new B(); obj.show(); } }