In C or C++, break and continue statements are the control statements that can change the flow of program execution.
The main difference between break and continue is, break statement is used to break the loop execution based on some conditional expression, whereas the continue statement skips the code that comes after it in a loop and begins a new iteration based on a certain condition.
Difference Between Continue And Break Statement in C++ in Tabular Form
Basis | Break Statement | Continue Statement |
---|---|---|
Definition | It is a control statement that is responsible for immediate termination of current enclosing loop (innermost loop in case of nested loop statements) | It is a control statement that is responsible for termination of current iteration of the loop and start next iteration again, without processing statements that come after it within the enclosing loop body |
Use | It is widely used in switch and loops | It is used in loops only |
Functionality | It terminates iteration of the loop or switch statement( in case of loops, it forces to exit from the loop and execute the code which immediate loop, in case of Switch it terminates the statement) | It skips the statements that come after it and goes for the next iteration of the loop |
Behaviour | When the break statement is encountered within the loop or switch statement, the execution is stopped immediately | When the continue statement is encountered within the loop during program execution, the statements after it is skipped and the control of loop jumps to the next iteration |
Termination | It causes early termination of the current loop | It causes an early execution of the next iteration of the loop |
Program flow | It typically stops the continuation of the current loop of the program | It only stops the current loop iteration but does not hampers the continuation of the loop in the program |
Break Statement
A break statement is a control statement that terminates the execution of a loop or a block in which it occurs. Therefore, it breaks the flow of a program.
In C++, the break statement is mainly used in these two cases :
a) Within a loop: When a break is triggered in a loop, it stops the loop execution immediately and transfers the control to the very next statement after it.
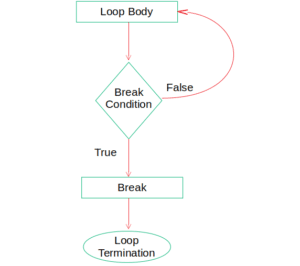
In the case of a nested loop, if a break is present in an inner loop, then it would terminate only the execution of that particular loop only.
b) In Switch Statement: In order to prevent consecutive execution of cases, every case in the switch statement comes with a break statement.
Syntax :
break ;
C++ program to show the working of Break Statement :
#include<iostream> using namespace std; int main() { for(int a=0;a<5;a++) {if(a==3) break; cout<<a<<" "; } return 0; }
Output :
0 1 2
Continue Statement
It only terminates the current iteration of the loop and starts the next iteration again, without processing statements that come after it within the enclosing loop body. It is used with looping statements only.
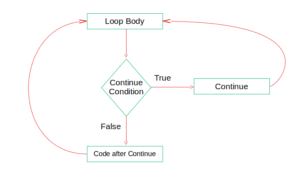
In simple words, it skips the current iteration and jumps to the next loop iteration without executing statements in the loop body that comes after it.
Syntax :
continue ;
C++ program to show the working of Continue Statement :
#include<iostream> using namespace std; int main() { for(int a=0;a<5;a++) { if(a==3) continue; cout<<a<<" "; } return 0; }
Output :
0 1 2 4