The program swaps two numbers. The functionality can be achieved in both ways by using or without using a temporary variable. The first approach uses temporary variable and second approach uses arithmetic operator.
Program to Swap two Numbers in C using a Temporary variable
In this approach, a temporary variable is used that acts as a container to hold a value for some time to swap two numbers. The code involves three variables.
Approach to swap two numbers :
- Store a value of first number to temporary variable.
- Then, assign the value of ‘ second variable’ to ‘first variable’.
- Lastly, put the value held by temporary variable (actually, value of first number) to ‘second number’.
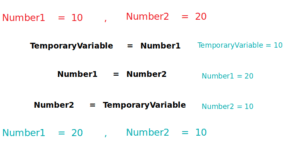
Program:
/* * Here's the program to swap two numbers in c using temp variable * */ #include <stdio.h> int main() { int number1, number2,temp; printf("\n Enter two numbers:\n First number = "); scanf("%d",&number1); printf(" Second number = "); scanf("%d",&number2); printf("\n\n ****** Before Swapping two numbers *******\n"); printf("\n First number = %d \n Second number = %d",number1,number2); temp=number1; number1=number2; number2=temp; printf("\n ********* After Swapping two numbers *****\n"); printf("\n First number = %d \n Second number = %d",number1,number2); return 0; }
Output:
Enter two numbers: First number = 6 Second number = 3 ****** Before Swapping two numbers******* First number = 6 Second number = 3 ********* After Swapping two numbers***** First number = 3 Second number = 6
Explanation
1) Getting two input numbers from the user, storing it to ‘number1’ and ‘number2’ respectively
2) Storing the first number(number1) to temp variable. for example, suppose numbere1 =3, number2=2, temp = 3
3) Storing value of “number2” to “number1” and value of “temp” to “number2”
5) As a result, both numbers are now swapped
Program to swap two numbers in C without using a temporary variable
In this approach, arithmetic operators are used which can be a combination of addition & subtraction and multiplication & division in order to swap two numbers. In this, we will be using addition & subtraction approach.
Approach to swap two numbers :
- Store the sum of two numbers to any of the given variable (suppose first variable ).
- Then, subtract other number from the sum and assign it to other variable (suppose second variable ).
- Subtract again and this time the outcome of second would be subtracted from the sum, and store it to first variable.
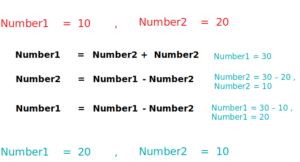
Program:
/* * Here's the program to swap two numbers in C without using temp variable * */ #include <stdio.h> int main() { int num,num1; printf("please enter the values : "); printf("\n First number = "); scanf("%d",&num); printf("\n Second number = "); scanf("%d",&num1); printf("\n Before Swapping two numbers........\n"); printf("\n first number = %d \n Second number = %d",num,num1); num = num +num1; num1=num-num1; num=num-num1; printf("\n After Swapping two numbers........\n"); printf("\n first number = %d \n Second number = %d",num,num1); return 0; }
Output:
please enter the values : First number = 89 Second number = 45 Before Swapping two numbers........ first number = 89 Second number = 45 After Swapping two numbers........ first number = 45 Second number = 89
Explanation
1) Getting two inputs from the user, storing it to ‘num’ and ‘num1’ variables respectively
2) Adding two numbers and storing it one of the variables ‘num’ or ‘num1’. for example, suppose num =3, num1=2, num = 3 +2 , num =5
3) Subtracting the num1 value from the result , num1 = num – num1 , num1 = 5- 2 = 3
4) Now, doing the same thing for num
5) As a result, both numbers are now swapped