Java supports a number of primitive data types or built-in types such as int, float, bool etc. variable which is followed by one of these primitive types can only store that corresponding value type.
In other words, if a variable is of float type, then it keeps float values only . Therefore, there is no sense of having methods.
Java provides a way to make a datatype to act as an object and this object keeps only these particular corresponding type of values, allowing these object to have useful methods to work with.
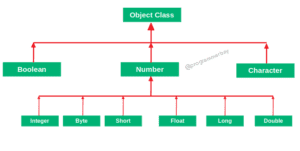
The concept of representing a primitive type as an object and the object that belongs to a class is known as Wrapper class.
For example, Integer, Float, Boolean are Wrapper classes. Wrapper classes are subclasses of abstract Number class.
There are some conversion operations among these wrapper classes and primitive type :
- Boxing
- Unboxing
- Autoboxing
- Autounboxing
1) Boxing: When a primitive type is converted to its corresponding wrapper class’s object explicitly, is known as Boxing.
int number= 6; Integer i=Integer.valueOf(number); // Boxing
2) Unboxing: When an object of the wrapper class is converted to primitive type explicitly, is known as Unboxing.
Integer i= new Integer(20); int number=i.intValue();// Unboxing
3) Autoboxing: When a primitive type is converted to its corresponding wrapper class’s object, implicitly by the compiler is known as Autoboxing.
int number= 6; Integer i=number; // Autoboxing
4) Autounboxing: When an object of the wrapper class is converted to primitive type, implicitly by the compiler is known as Autounboxing.
Integer i= new Integer(20); int number=i; // Autounboxing
Java program to demonstrate the working Integer Wrapper Class
Program:
public class WrapperClass { public static void main (String[]args) { int intVar1 = 8; int intVar2 = 20; Integer number1 = new Integer (intVar1); Integer number2 = new Integer (intVar2); System.out.println ("****** byteValue()********"); // returns byte equivalent value System.out.println ("Getting byte value using bytevalue() = " + number1.byteValue ()); System.out.println ("\n******CompareTo()********"); // if it returns 0, then the numbers are same. //if it returns negitive number, then calling object would be smaller than other. //if it returns positive number, then calling object would be larger than other. System.out.println ("Comparing two Integer Objects = " + number1.compareTo (number2)); System.out.println ("\n****** doubleValue()********"); // returns value of double type which is correspoding to integer Object System.out.println ("Double type of this object = " + number1.doubleValue ()); System.out.println ("\n****** equals()********"); // returns true, if two objects are same, otherwise false System.out.println ("Comparig two objects to check whether they are equal or not = " + number1.equals (number2)); System.out.println ("\n****** toString()********"); // returns String object identical to integer value System.out.println ("String object = " + number1.toString ()); } }
Output: